Predicting with a Neural Network explained
text
Predicting with a Neural Network
In this post, we'll be discussing what it means for an artificial neural network to predict, and we'll also see how to do predictions in code using Keras.
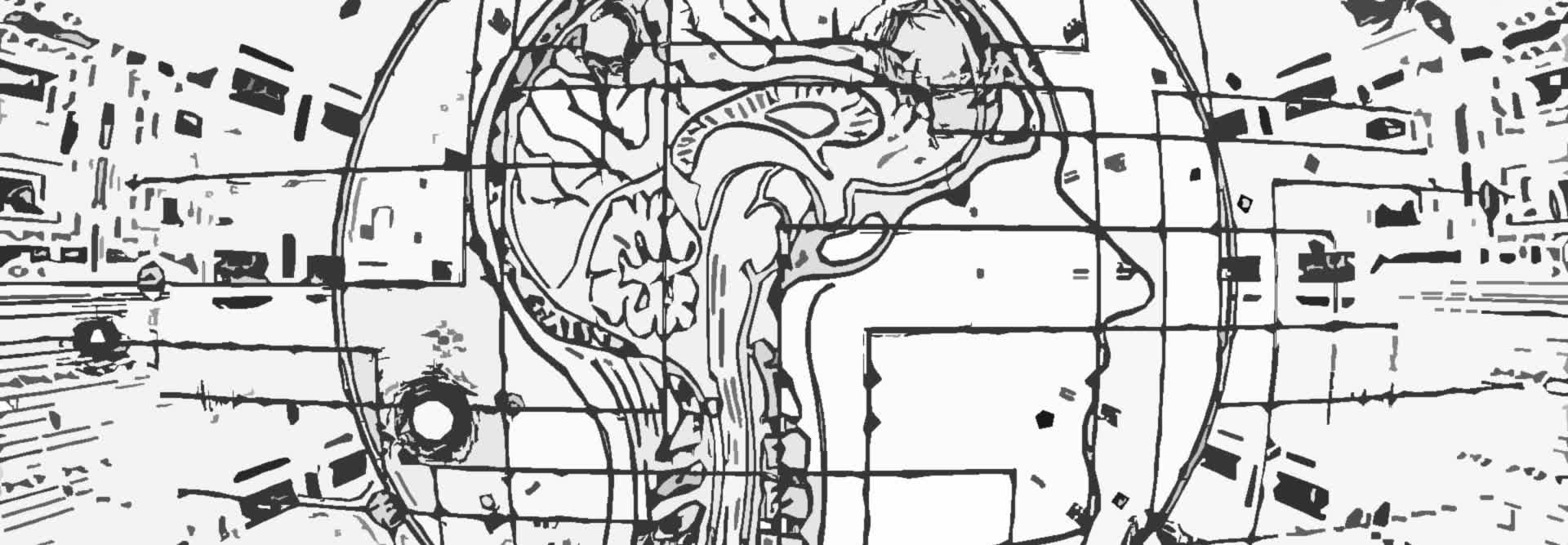
In an earlier post, we discussed what it means to train a neural network. After this training has completed, if we're happy with the metrics that the model gave us for our training and validation data, then the next step would be to have our model predict on the data in our test set.
Recall from our post on training, testing, and validation sets, that unlike the train and validation data that get passed to the model with their respective labels, when we pass our test data to the model, we do not pass the corresponding labels. So, the model is not aware of the labels for the test set at all.
Passing samples with no labels
For predicting, essentially what we're doing is passing our unlabeled test data to the model and having the model predict on what it thinks about each sample in our test data. These predictions are occurring based on what the model learned during training.
For example, suppose we trained a model to classify different breeds of dogs based on dog images. For each sample image, the model outputs which breed it thinks is most likely.
Now, suppose our test set contains images of dogs our model hasn't seen before. We pass these samples to our model, and ask it to predict the output for each image. Remember, the model does not have access to the labels for these images.
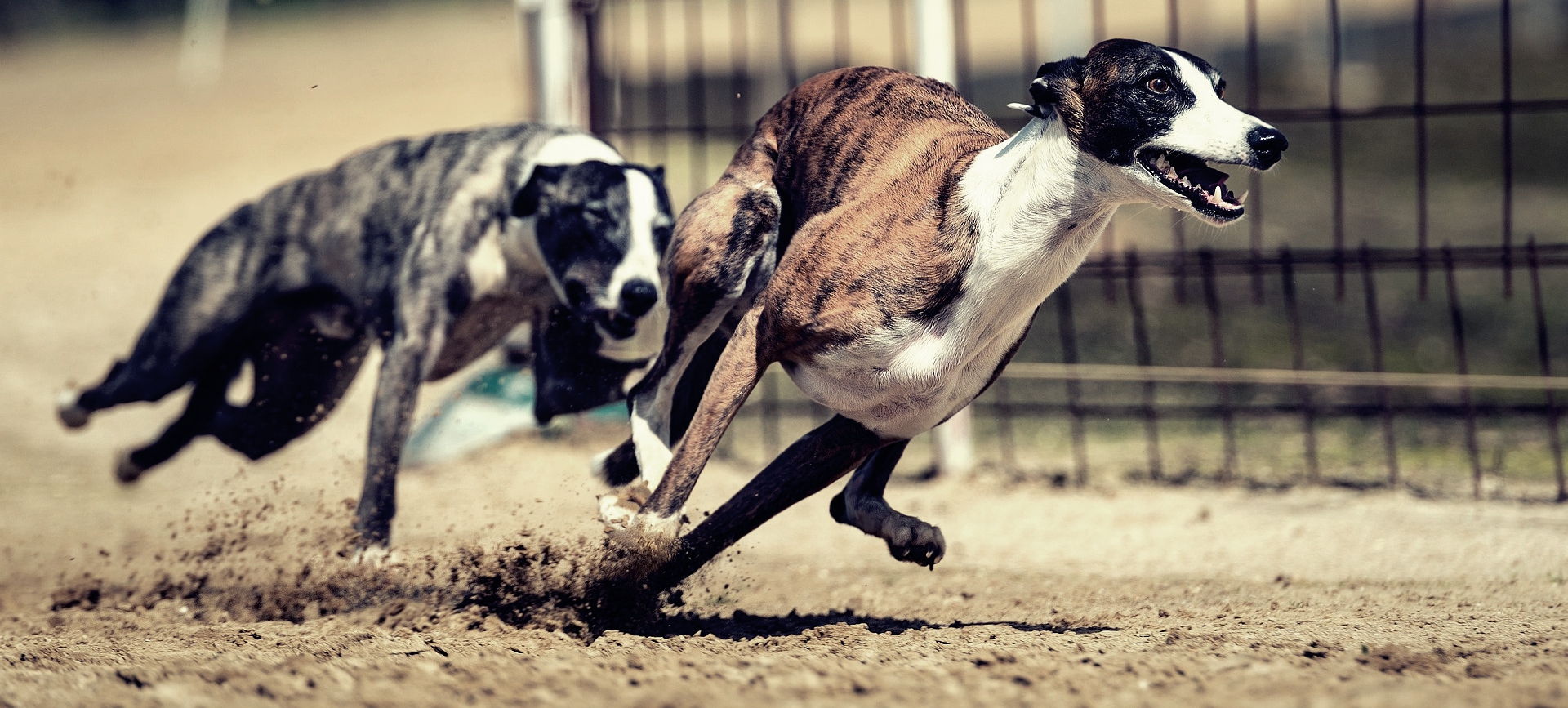
This process will tell us how well our model performs on data it hasn't seen before based on how well its predictions match the true labels for the data.
This process will also help give us some insight on what our model has or hasn't learned. For example, suppose we trained our model only on images of large dogs, but our test set has some images of small dogs. When we pass a small dog to our model, it likely isn't going to do well at predicting what breed the dog is, since it's not been trained very well on smaller dogs in general.
This means that we need to make sure that our training and validation sets are representative of the actual data we want our model to be predicting on.
Note that it is possible to pass unlabeled data to our model for predictions prior to training, however, we would expect the model to perform poorly with these predictions since it has not yet learned how to classify the data in the training set.
Deploying the model in the real world (production)
Aside from running predictions on our test data, we can also have our model predict on real world data once it's deployed to serve its actual purpose.
For example, if we deployed this neural network for classifying dog breeds to a website that anyone could visit and upload an image of their dog, then we'd want to be predicting the breed of the dog based on the image.
This image would likely not have been one that was included in our training, validation, or test sets, so this prediction would be occurring with true data from out in the field.
Let's see now how we can do these predictions in code using Keras.
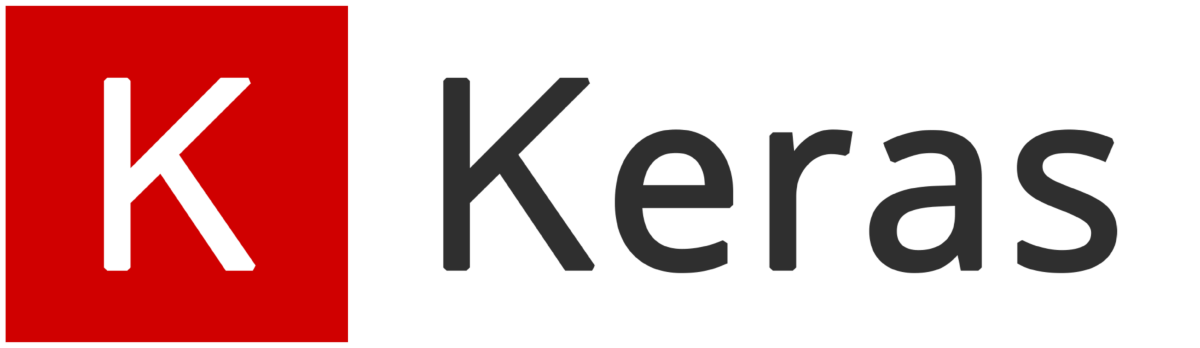
Using a Keras model to get a prediction
Suppose we have the following code:
predictions = model.predict(
x=scaled_test_samples,
batch_size=10,
verbose=0
)
The first item we have here is a variable we've called predictions
. We're assuming that we already have our model built and trained. Our model in this example is the object called
model
. We're setting predictions
equal to model.predict()
.
This predict()
function is what we call to actually have the model make predictions. To the predict()
function, we're passing the variable called
scaled_test_samples
. This is the variable that's holding our test data.
We set our batch_size
here arbitrarily to 10
. We set the verbosity, which is how much we want to see printed to the screen when we run these predictions, to 0
here
to show nothing.
Before going forward, note that we are just using a sample model here that we've used in previous posts. We won't go into any details about the actual model now, but if you're interested in building the same model and running these same predictions, then check out the posts from the Keras series on preprocessing data and creating a confusion matrix. They will give you the full picture regarding this test data.
For now, we're just showing the concept of how to run predictions in code with Keras.
Ok, so we ran our predictions. Now let's look at our output.
for p in predictions:
print(p)
[ 0.7410683 0.2589317]
[ 0.14958295 0.85041702]
...
[ 0.87152088 0.12847912]
[ 0.04943148 0.95056852]
For this sample model, we have two output categories, and we're just printing each prediction from each sample in our test set, which is stored in our predictions
variable.
We see we have two columns here. These represent the two output categories, and are showing us probabilities for each category. These are the actual predictions. Let's call the categories
0
and 1
for simplicity.
For example, for the first sample in our test set, the model is assigning a 74%
probability that the sample falls into category 0
and only a 26%
probability that it
falls into category 1
.
The second sample shows us that the model is assigning an 85%
probability to the sample being in category 1
, and a 15%
probability that it's in category
0
, and this occurs for each of the test samples in our predictions variable.
Wrapping up
That's really all there is to it to having a neural network make predictions in Keras! Hopefully now we have an understanding of what it means to have a neural network predict on data. I'll see you in the next one!
quiz
resources
updates
Committed by on