Neural Network Predictions with TensorFlow's Keras API
text
Neural network predictions with TensorFlow's Keras API
In this episode, we'll demonstrate how to use a neural network for inference to make predictions on data from a test set. We'll continue working with the same tf.keras.Sequential
model and data that we've used in the last few episodes to do so.
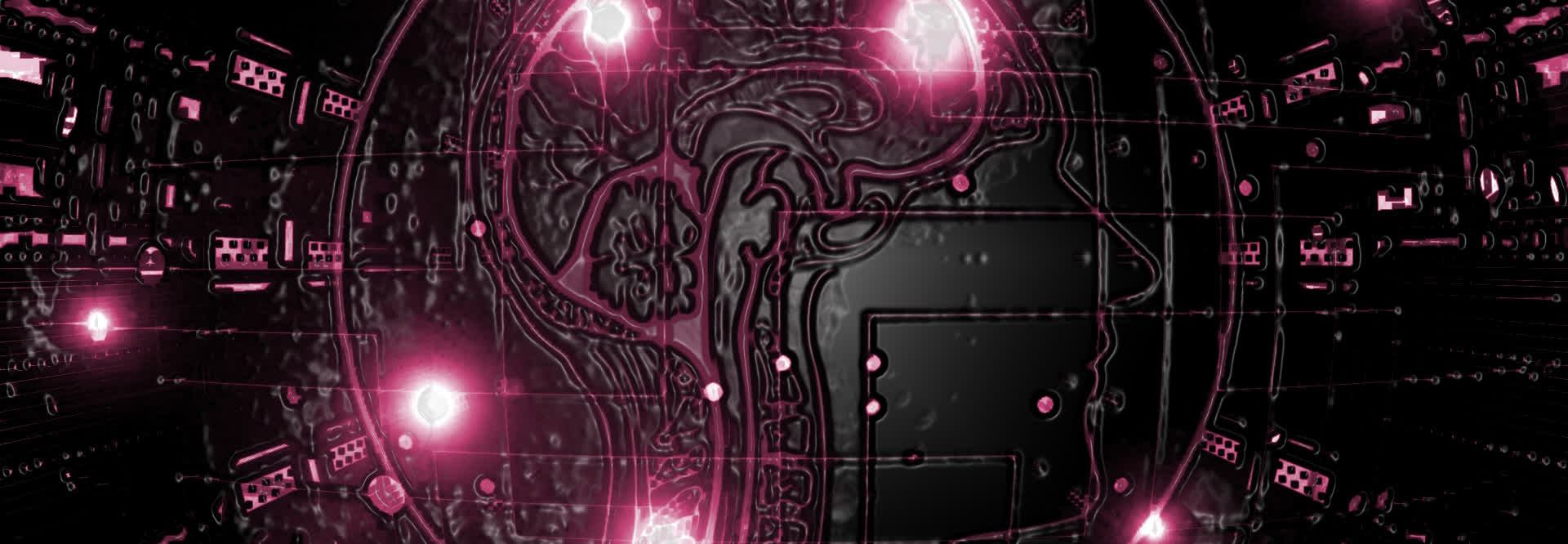
As we touched on previously, when we train a model, the hope is that we'll later be able to take the trained model, apply it to new data, and have the model generalize and accurately predict on data it hasn't seen before.
For example, suppose we have a model that categorizes images of cats or dogs and that the training data contained thousands of images of cats and dogs from a particular data set online.
What is Inference?
Now suppose that later we want to take this model and use it to predict on other images of cats and dogs from a different data set. The hope is that, even though our model wasn't exposed to these particular dog and cat images during training, it will still be able to accurately make predictions for them based on what it's learned from the cat and dog data set from which it was trained.
We call this process inference, as the model is using its knowledge gained from training and using it to infer a prediction or result.
At this point, the model we've been working with over the past few episodes has now been trained and validated. Given the results we've seen from the validation data, it appears that this model should do well on predicting on a new test set.
Note that the test set is the set of data used specifically for inference after training has concluded. You can find more information on all the different types of data sets used in deep learning in the Deep Learning Fundamentals course.
Creating the test set
We'll create a test set in the same fashion for which we created the training set. In general, the test set should always be processed in the same way as the training set.
We won't go step-by-step over the code that generates and processes the test data below, as it has already been covered in detail in an earlier episode where we generated the training data, but be sure you have all the imports in place from the previous episodes, as well as all of the existing code up to this point.
test_labels = []
test_samples = []
for i in range(10):
# The 5% of younger individuals who did experience side effects
random_younger = randint(13,64)
test_samples.append(random_younger)
test_labels.append(1)
# The 5% of older individuals who did not experience side effects
random_older = randint(65,100)
test_samples.append(random_older)
test_labels.append(0)
for i in range(200):
# The 95% of younger individuals who did not experience side effects
random_younger = randint(13,64)
test_samples.append(random_younger)
test_labels.append(0)
# The 95% of older individuals who did experience side effects
random_older = randint(65,100)
test_samples.append(random_older)
test_labels.append(1)
test_labels = np.array(test_labels)
test_samples = np.array(test_samples)
test_labels, test_samples = shuffle(test_labels, test_samples)
scaled_test_samples = scaler.fit_transform(test_samples.reshape(-1,1))
Note that the MinMaxScaler
object scaler
we're making use of at the end of this code block was defined in a
previous episode.
Evaluating the test set
To get predictions from the model for the test set, we call model.predict()
.
predictions = model.predict(
x=scaled_test_samples
, batch_size=10
, verbose=0
)
To this function, we pass in the test samples x
, specify a batch_size
, and specify which level of verbosity we want from log messages during prediction generation. The output from
the predictions won't be relevant for us, so we're setting verbose=0
for no output.
Note that, unlike with training and validation sets, we do not pass the labels of the test set to the model during the inference stage.
To see what the model's predictions look like, we can iterate over them and print them out.
for i in predictions:
print(i)
[ 0.74106830 0.25893170]
[ 0.14958295 0.85041702]
[ 0.96918124 0.03081879]
[ 0.12985019 0.87014979]
[ 0.88596725 0.11403273]
...
Each element in the predictions
list is itself a list of length 2
. The sum of the two values in each list is 1
. The reason for this is because the two columns contain
probabilities for each possible output:
experienced side effects and
did not experience side effects. Each element in the predictions
list is a probability distribution over all possible outputs.
The first column contains the probability for each patient not experiencing side effects, which is represented by a 0
. The second column contains the probability for each patient experiencing
side effects, which is represented by a 1
.
We can also look only at the most probable prediction.
rounded_predictions = np.argmax(predictions, axis=-1)
for i in rounded_predictions:
print(i)
0
1
0
1
0
...
From the printed prediction results, we can observe the underlying predictions from the model, however, we cannot judge how accurate these predictions are just by looking at the predicted output.
If we have corresponding labels for the test set, (for which, in this case, we do), then we can compare these true labels to the predicted labels to judge the accuracy of the model's evaluations. We'll see how to visualize this using a tool called a confusion matrix in the next episode.
quiz
resources
updates
Committed by on