Keras with TensorFlow Prerequisites - Getting Started With Neural Networks
text
Course description
In this course, we will learn how to use Keras, a neural network API written in Python! Each episode focuses on a specific concept and shows how the full implementation is done in code using Keras and Python.
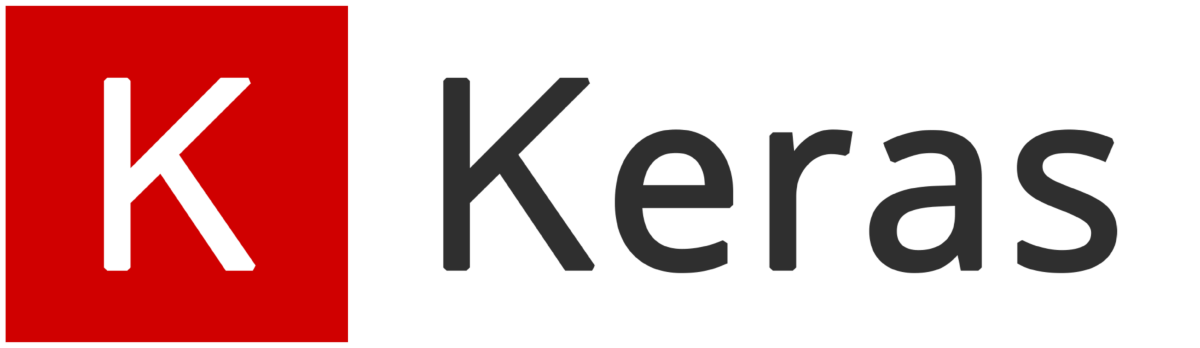
We'll be starting from the basics by learning how to organize and preprocess data, and then we'll move on to building and training artificial neural networks.
Some of the networks we'll build from scratch, and others will be pre-trained state-of-the-art models that we'll fine-tune to our data. After getting comfortable with building and training networks, we'll learn how to deploy our models using both front-end and back-end deployment techniques.
Prerequisites
From a knowledge standpoint, many deep learning concepts will be explained and covered as we progress through this course, however, if you are brand new to deep learning, then it is recommended that you start with our Deep Learning Fundamentals course first.
The Deep Learning Fundamentals course will teach you everything you need to know to get acquainted with all the major deep learning concepts. You can then take your newly gained knowledge from that course, and come to apply it in this Keras course.
If you're eager to jump into the code, then you can choose to take both courses simultaneously.
In regards to coding prerequisites, just some basic coding skills and Python experience are needed!
Course Resources
This course has plenty of resources to ensure your success.
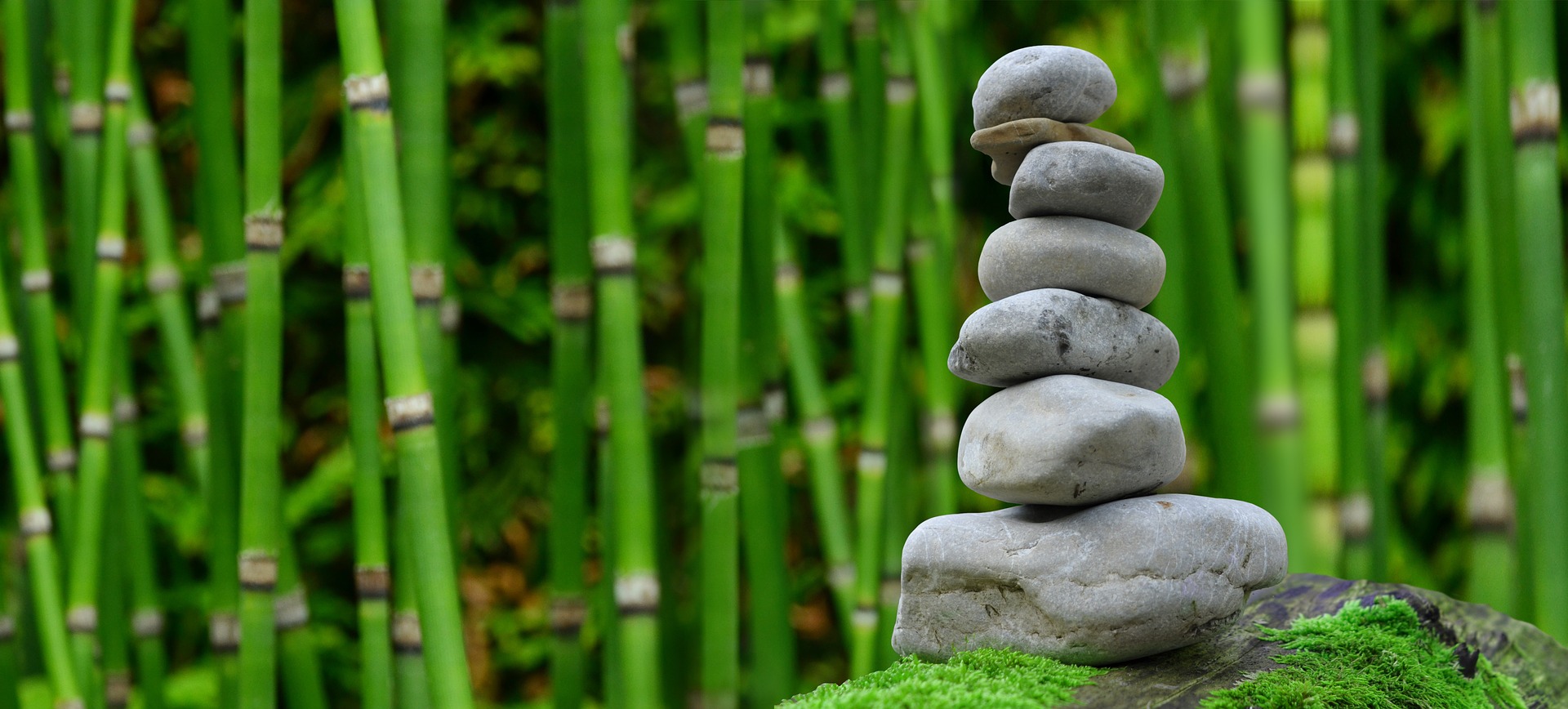
Here is a list of resources you'll have right at your fingertips.
- Text and video resources on deeplizard.com
- Create your own quiz questions to be featured on deeplizard.com
- Question and answer comments by the community on YouTube
- Regularly tested and maintained code providing updates and fixes
- Download access to code files available for the deeplizard hivemind
Why Keras?
Keras was developed with a focus on enabling fast experimentation. Because of this, it's very user friendly and allows us to go from idea to implementation with only a few steps.
Aside from this, users often wonder why choose Keras over another neural network API?
Our general advice is to learn more than one neural network API. Once you have the core deep learning concepts down from the Deep Learning Fundamentals course, you can implement your knowledge in code across several APIs.
Yes, the syntactical details for implementation will differ slightly between APIs, but once you learn one, it is relatively easy to catch on to another. Sometimes, one API may have an advantage over the others for a particular implementation.
Especially for job prospects, knowing more than one API will put more tools in your skill set, and demonstrating this will make you a more valuable candidate.
TensorFlow Integration
Keras was originally created by FranΓ§ois Chollet. Historically, Keras was a high-level API that sat on top of one of three lower level neural network APIs and acted as a wrapper to to these lower level libraries. These libraries were referred to as Keras backend engines.
You could choose TensorFlow, Theano, or CNTK as the backend engine you'd like to work with.
- TensorFlow
- Theano
- CNTK
Ultimately, TensorFlow became the most popular backend engine for Keras.
Later, Keras became integrated with the TensorFlow library and now comes completely packaged with it.
Now, when you install TensorFlow, you also automatically get Keras, as it is now part of the TensorFlow library.
Differences in Imports
From a usability standpoint, many changes between the older way of using Keras with a configured backend versus the new way of having Keras integrated with TensorFlow is in the import statements.
For example, previously, we could access the Dense
module from Keras with the following import statement.
from keras.layers import Dense
Now, using Keras with TensorFlow, the import statement looks like this:
from tensorflow.keras.layers import Dense
Below, you can see the difference between the old way and new way of importing some common Keras modules.
Before TensorFlow Integration
import keras
from keras.models import Sequential
from keras.layers import Activation
from keras.layers.core import Dense
from keras.optimizers import Adam
from keras.metrics import categorical_crossentropy
from keras.preprocessing.image import ImageDataGenerator
from keras.layers.normalization import BatchNormalization
from keras.layers.convolutional import Conv2D
After TensorFlow Integration
import tensorflow
from tensorflow import keras
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Activation, Dense, BatchNormalization, Conv2D
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.metrics import categorical_crossentropy
from tensorflow.keras.preprocessing.image import ImageDataGenerator
How to Install Keras
Since Keras now comes packaged with TensorFlow, we need to install TensorFlow with the command:
pip install tensorflow
That's it!
Be sure to stay up to date with any code or software changes in the future, which will be correspondingly fixed and updated on deeplizard.com/resources.
Syllabus
Below is the syllabus for the course:
- Part 1: Artificial Neural Network Basics
- Section 1: Intro to Keras and neural networks
- Processing data
- Building and training neural networks
- Validation and inference
- Saving and loading models
- Section 2: Convolutional Neural Networks (CNNs)
- Image processing
- Building and training CNNs
- Using CNNs for inference
- Section 3: Fine-tuning and transfer learning
- Intro to fine-tuning and VGG16 model
- Implement fine-tuning on VGG16 model
- Using fine-tuned models for inference
- Intro to MobileNet
- Fine-tuning MobileNet on custom data set
- Section 4: Additional topics
- Data augmentation
- Keras' image labeling implementation
- Achieving reproducible results
- Learnable parameters
- Part 2: Neural network model deployment
- Section 1: Deployment with Flask
- Introduction to Flask and web services
- Build a simple Flask app and web app
- Send and receive data with Flask
- Host neural network with Flask
- Build neural network web app to interact with Flask service
- Integrating data visualization with D3, DC, Crossfilter
- Alternative ways to access neural network from Powershell and Curl
- Information privacy and data protection
- Section 2: Deployment with TensorFlow.js
- Introduction to client-side neural networks
- Convert Keras model to TFJS model
- Set up Node.js and Express
- Build UI for neural network web app
- Host a neural network with TFJS
- Explore tensor operations through image processing
- Examine tensor operations with debugger
- Broadcasting tensors
- Efficiency of hosting MobileNet in the browser
Looking forward to hearing from you in the course! Let's get started!
quiz
resources
updates
Committed by on